Wednesday, March 22, 2006
Natural disasters aren't disasters... unless they involve living or monetary things.
Friday, March 17, 2006
Diesel, successor to the language Cecil
http://wasp.cs.washington.edu/wasp_diesel.html
Lots of good ideas and I respect the work done on predicate dispatching, but I think the language could have been simplified. Also, using reflection with pure multimethod dispatching is tricky. Can you ask an object which methods it supports?
Lots of good ideas and I respect the work done on predicate dispatching, but I think the language could have been simplified. Also, using reflection with pure multimethod dispatching is tricky. Can you ask an object which methods it supports?
Wednesday, March 15, 2006
Paragraphs with half-spaced newlines
I've been working on my Inertia UI ideas again, this time in Ruby. I was walking home from the train today and remembered an idea I had for a code editor: paragraphs with half spaced newlines, i.e. blank lines that are only half height. You know sometimes when you want to space out your code, but a full newline is just too big? Bingo.
class Button < View
attr :caption, true
attr :action, true
# ---------------------------------- #
def initialize()
super
self.extent = [100, 25]
self.caption = "Click Me!"
end
# ---------------------------------- #
def mouse_up( origin )
action.call() if action
end
end
Monday, March 13, 2006
Clever Ruby 2.0? enumerations
Ok, so you can iterate over a collection:
(1..10).each { |v, i| puts v * i }And you can iterate over a collection with an index:
(1..10).each_with_index { |v, i| puts v * i }But if you want map_with_index, select_with_index, etc. it causes an explosion of methods to create. What if each is called without a block, and passed along to the next enumerator:
(1..10).each.with_index { |v, i| puts v * i }Nice! Why? Because now you can do things like:
(1..10).collect.with_index { |v, i| v * i }without pre-defining this functionality. It's more general, has the same number of keystrokes, and the syntax is only a one character difference. More info here
Sunday, March 12, 2006
Many solutions, different efficiencies
I compared 3 ways of creating even-squares of numbers in Ruby, and the fastests seems to be 'map {} .compact!'. Here are the methods, each performed 1,000,000 times:
1: (1..10).inject( Array.new )and here are the results:
{ |a, i| a << i * i if i % 2 == 0; a }
2: (1..10).select { |i| i % 2 == 0 }.collect { |i| i * i }
3: (1..10).map { |i| i * i if i % 2 == 0 }.compact!
user system total realThe first one is probably the most memory efficient, while the last is probably the least memory efficient. Fast, Cheap, Good - pick *two* ;)
1: 31.734000 0.015000 31.749000 ( 31.956000)
2: 22.313000 0.000000 22.313000 ( 22.416000)
3: 19.890000 0.000000 19.890000 ( 19.976000)
Music Based on Fibonacci Sequence and Stock Market
If you haven't already seen it on Slashdot,
Music Based on Fibonacci Sequence and Stock Market
Music Based on Fibonacci Sequence and Stock Market
Friday, March 10, 2006
Quote of the week...
An engineer is someone who will spend two hours debating the probable outcome of a five minute experiment.
~ Unknown
Tuesday, March 07, 2006
When developers design...
I've just downloaded VisualWorks Smalltalk 7.4 non-commercial, and I have to say that well, it looks like the IDE was designed by programmers. Many portions of the environment are confusing, and the terminology is different than what Mac/PC coders are used to - you don't 'save' and interface, you 'install' it; and you don't 'run' it to test it, you 'open' it. I'm still trying to figure out how to make a button open a window and say 'hello world'. Smalltalk is such a simple, beautiful language - I wish there were an environment with those same properties.
And here's a curious message I recently encountered:
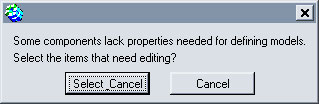
There are just too many terms that sound similar: Namespaces, Packages, Parcels and Bundles. Also, I can't seem to create a new method for a class. I can create a new class and protocol, but there is no 'new method'?? This is a rant, if you couldn't tell. I just wonder sometimes why things have to be so difficult.
And here's a curious message I recently encountered:
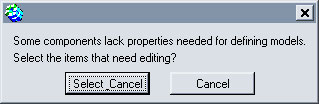
There are just too many terms that sound similar: Namespaces, Packages, Parcels and Bundles. Also, I can't seem to create a new method for a class. I can create a new class and protocol, but there is no 'new method'?? This is a rant, if you couldn't tell. I just wonder sometimes why things have to be so difficult.
Sunday, March 05, 2006
Very different languages
Here's one way to find prime numbers in Ruby:
http://www.kx.com/a/k/examples/xml.k
Interesting for cranking out ad-hoc formulas maybe. But writing programs in it? I don't know about that. But if you want to learn more about it, visit A Shallow Introduction to the K Programming Language
def isprime(n)And here it is translated into the language "K":
(2..n-1).map {|x| n % x}.min
end
isprime: {&/x!/:2_!x}Here's a step by step breakdown:
!7 enumerate 7, i.e. 0..6If you really want to hurt your eyes, see a larger example:
2_ (!7) drop first 2 elements
: (2_ (!7)) set value of
7 !/ (: (2_ (!7))) vector modulus
&/ (7 !/ (: (2_ (!7)))) vector minimum
http://www.kx.com/a/k/examples/xml.k
Interesting for cranking out ad-hoc formulas maybe. But writing programs in it? I don't know about that. But if you want to learn more about it, visit A Shallow Introduction to the K Programming Language